写在最前面
项目完整源码(欢迎star):https://github.com/wjup/shorturl
引入主题
前几天在某论坛看到这样一篇帖子,说的是“大家第一个项目,都是从网址导航开始?”,浏览半天回复的内容发现都是大佬啊,有做了个浏览器插件的,有做了个博客的,这个更不错,手撸出来的多厉害。当然也有不少做的网址导航的。
翻到上面看到一位老哥说的是短网址项目。

于是我脑瓜一热,百度了下短网址的原理。发现还是很简单的,说时迟那时快,我已经打开了IDEA,创建项目手撸开干。项目架构选择了springboot,持久层还是用的mybatis,前端依然是bootstrap。
用了不到一个下午的时间项目已经出来了。整个项目中,最浪费时间的还是前端调样式。前端菜的一批。
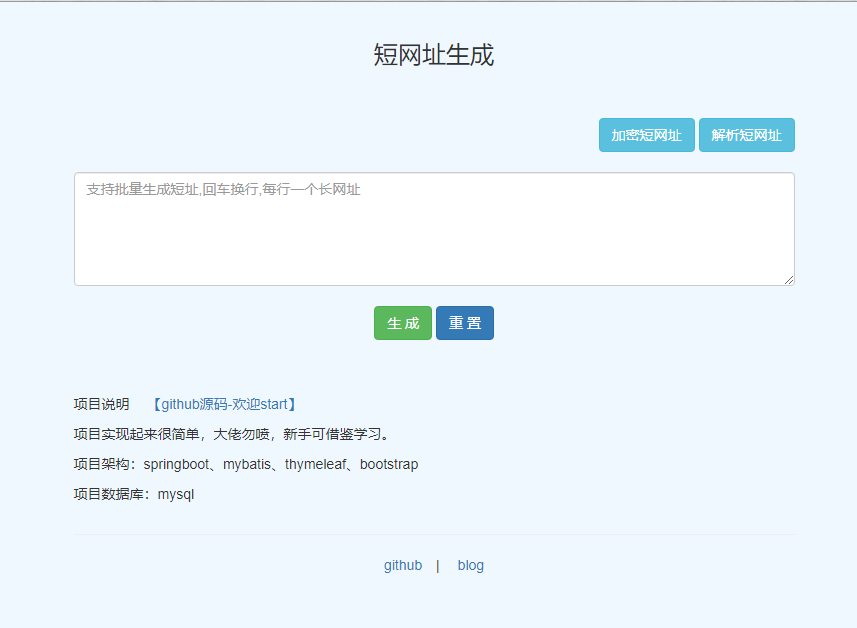
实现原理
下面说下实现的原理
首先在用户输入一条长链接传到后端的时候,我先生成了数字和字母随机组成的6位字符,然后把这个字符和长链接保存到数据库。保存成功后,把这6位字符拼上我的网址,返回给用户,就像是这样的 https://wjup.top/HtN3Gc
当用户拿到这个短网址访问的时候。我在后台进行获取这个短网址的6个字符,然后根据这个字符到数据库查询原来的链接,再进行301永久重定向到原网址就可以了。整体实现非常简单。当然我还增加了对短网址加密的功能,只有输入正确的密码才能访问原始链接
逻辑代码
下面放出主要的实现代码。项目完整版可以到github中去克隆岛到本地研究
1 | package com.wjup.shorturl.controller; |