简单的说WebSocket最大的好处就是:不用重复刷新,就可以实现前后台不断交互
什么是WebSocket?
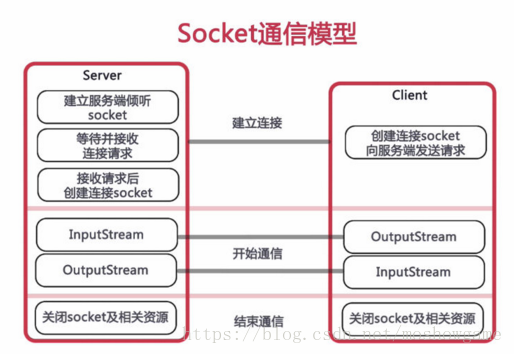
WebSocket协议是基于TCP的一种新的网络协议。它实现了浏览器与服务器全双工(full-duplex)通信——允许服务器主动发送信息给客户端。
为什么需要 WebSocket?
初次接触 WebSocket 的人,都会问同样的问题:我们已经有了 HTTP 协议,为什么还需要另一个协议?它能带来什么好处?
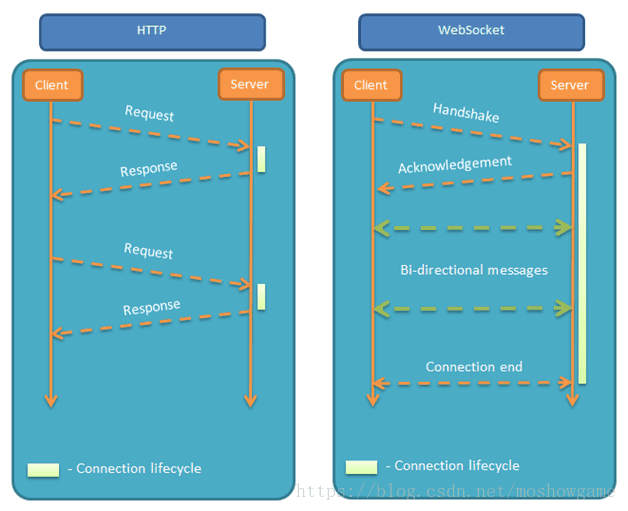
答案很简单,因为 HTTP 协议有一个缺陷:通信只能由客户端发起,HTTP 协议做不到服务器主动向客户端推送信息。
举例来说,我们想要查询当前的排队情况,只能是页面轮询向服务器发出请求,服务器返回查询结果。轮询的效率低,非常浪费资源(因为必须不停连接,或者 HTTP 连接始终打开)。因此WebSocket 就是这样发明的。
maven依赖
1 | <dependency> |
WebSocketConfig
启用WebSocket的支持也是很简单,几句代码搞定
1 | import org.springframework.context.annotation.Bean; |
WebSocketServer
因为WebSocket是类似客户端服务端的形式(采用ws协议),那么这里的WebSocketServer其实就相当于一个ws协议的Controller
直接@ServerEndpoint(“/websocket”)@Component启用即可,然后在里面实现@OnOpen,@onClose,@onMessage等方法
1 | import java.io.IOException; |
消息推送
至于推送新信息,可以再自己的Controller写个方法调用WebSocketServer.sendInfo();即可
1 |
|
页面发起socket请求
然后在页面用js代码调用socket,当然,太古老的浏览器是不行的,一般新的浏览器或者谷歌浏览器是没问题的。还有一点,记得协议是ws的哦,如果像我这样封装了一些basePath的路径类,可以replace(“http”,“ws”)来替换协议
1 | var socket; |
js获取路径
获取主机名
1 | var hostname = location.hostname; |
获取端口号
1 | var port = location.port; |
获取主机名+端口号
1 | var host = location.host; |
访问:172.16.0.1:30/login.html
1 | hostname = 172.16.0.1; |